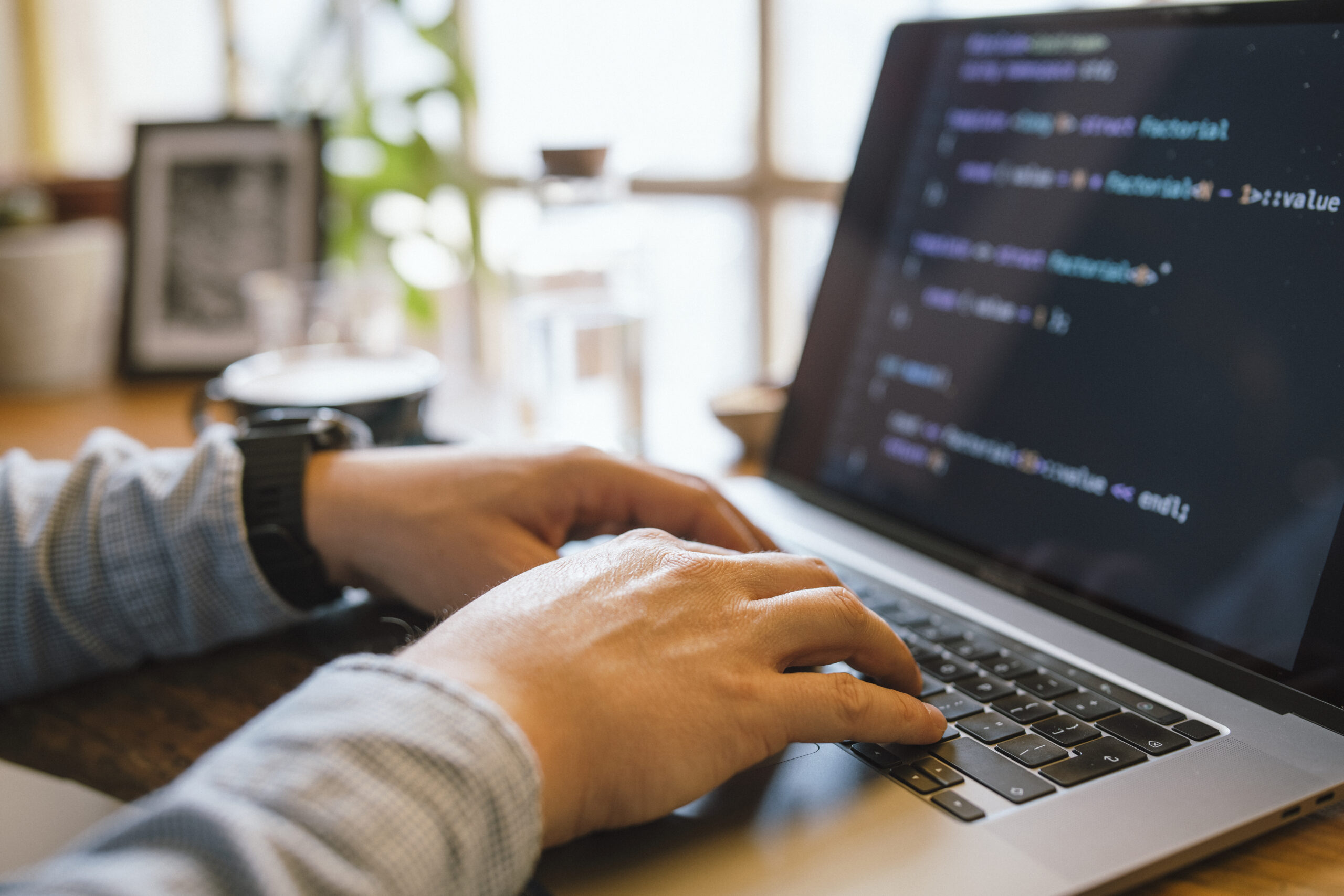
Debugging is The most essential — but generally missed — skills inside a developer’s toolkit. It is not nearly repairing broken code; it’s about comprehension how and why points go Completely wrong, and Discovering to think methodically to solve problems efficiently. Regardless of whether you're a newbie or perhaps a seasoned developer, sharpening your debugging abilities can conserve hours of aggravation and significantly enhance your productivity. Here are several strategies that will help builders stage up their debugging recreation by me, Gustavo Woltmann.
Master Your Tools
On the list of fastest approaches developers can elevate their debugging skills is by mastering the applications they use everyday. Even though composing code is 1 part of enhancement, figuring out the way to interact with it correctly through execution is equally important. Modern-day advancement environments come Geared up with effective debugging capabilities — but quite a few developers only scratch the floor of what these resources can perform.
Just take, for instance, an Built-in Advancement Environment (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These resources allow you to established breakpoints, inspect the value of variables at runtime, action via code line by line, and perhaps modify code within the fly. When used accurately, they let you notice exactly how your code behaves for the duration of execution, that is priceless for monitoring down elusive bugs.
Browser developer equipment, which include Chrome DevTools, are indispensable for front-conclusion developers. They enable you to inspect the DOM, monitor network requests, watch genuine-time effectiveness metrics, and debug JavaScript within the browser. Mastering the console, sources, and community tabs can turn annoying UI challenges into manageable duties.
For backend or procedure-level developers, instruments like GDB (GNU Debugger), Valgrind, or LLDB give deep Handle about running processes and memory management. Mastering these tools could have a steeper Mastering curve but pays off when debugging performance problems, memory leaks, or segmentation faults.
Over and above your IDE or debugger, develop into comfortable with version Handle programs like Git to be familiar with code history, discover the exact second bugs have been launched, and isolate problematic improvements.
In the end, mastering your equipment signifies heading outside of default configurations and shortcuts — it’s about acquiring an personal expertise in your development environment to ensure that when problems come up, you’re not misplaced at midnight. The better you realize your resources, the more time you are able to invest solving the particular trouble rather then fumbling as a result of the procedure.
Reproduce the situation
Among the most important — and sometimes neglected — measures in successful debugging is reproducing the issue. Prior to leaping in the code or generating guesses, developers need to produce a reliable setting or situation where the bug reliably seems. With no reproducibility, fixing a bug becomes a video game of possibility, frequently bringing about squandered time and fragile code modifications.
The initial step in reproducing a difficulty is gathering just as much context as you can. Ask issues like: What actions led to The problem? Which atmosphere was it in — enhancement, staging, or creation? Are there any logs, screenshots, or error messages? The greater detail you may have, the less difficult it results in being to isolate the exact conditions underneath which the bug happens.
Once you’ve collected enough data, attempt to recreate the situation in your local setting. This could indicate inputting the same knowledge, simulating similar consumer interactions, or mimicking procedure states. If the issue seems intermittently, think about producing automated assessments that replicate the sting circumstances or point out transitions involved. These exams not simply help expose the challenge but will also stop regressions Sooner or later.
Sometimes, the issue could possibly be ecosystem-particular — it would materialize only on certain working devices, browsers, or less than specific configurations. Employing instruments like Digital machines, containerization (e.g., Docker), or cross-browser testing platforms could be instrumental in replicating these kinds of bugs.
Reproducing the condition isn’t simply a step — it’s a attitude. It calls for endurance, observation, in addition to a methodical approach. But when you can constantly recreate the bug, you are previously midway to repairing it. That has a reproducible circumstance, You may use your debugging tools more successfully, check prospective fixes securely, and talk a lot more Obviously along with your group or consumers. It turns an abstract complaint right into a concrete obstacle — Which’s where by builders prosper.
Read through and Recognize the Error Messages
Error messages tend to be the most respected clues a developer has when something goes wrong. Rather then looking at them as discouraging interruptions, developers ought to discover to take care of mistake messages as direct communications in the system. They normally inform you just what happened, where by it took place, and often even why it happened — if you know the way to interpret them.
Start off by reading through the message thoroughly and in full. Lots of developers, especially when underneath time stress, look at the primary line and instantly get started building assumptions. But deeper during the error stack or logs may perhaps lie the real root trigger. Don’t just duplicate and paste error messages into search engines like google and yahoo — read through and comprehend them initially.
Break the mistake down into components. Can it be a syntax error, a runtime exception, or possibly a logic error? Does it issue to a particular file and line selection? What module or operate brought on it? These queries can guideline your investigation and level you towards the responsible code.
It’s also valuable to understand the terminology on the programming language or framework you’re using. Error messages in languages like Python, JavaScript, or Java generally follow predictable designs, and Discovering to recognize these can substantially increase your debugging procedure.
Some errors are vague or generic, As well as in Those people instances, it’s critical to look at the context in which the error transpired. Look at associated log entries, input values, and up to date improvements in the codebase.
Don’t neglect compiler or linter warnings both. These normally precede larger concerns and supply hints about probable bugs.
Finally, error messages aren't your enemies—they’re your guides. Finding out to interpret them effectively turns chaos into clarity, encouraging you pinpoint issues more quickly, lessen debugging time, and turn into a far more successful and self-assured developer.
Use Logging Wisely
Logging is Probably the most effective equipment in the developer’s debugging toolkit. When utilised properly, it offers true-time insights into how an software behaves, supporting you fully grasp what’s occurring beneath the hood while not having to pause execution or phase throughout the code line by line.
An excellent logging method begins with realizing what to log and at what degree. Typical logging ranges consist of DEBUG, INFO, Alert, Mistake, and Deadly. Use DEBUG for in depth diagnostic info throughout development, Facts for typical gatherings (like prosperous start off-ups), WARN for potential challenges that don’t split the appliance, ERROR for precise troubles, and Deadly when the procedure can’t keep on.
Stay away from flooding your logs with excessive or irrelevant details. An excessive amount logging can obscure crucial messages and slow down your process. Target important situations, condition modifications, input/output values, and important selection points as part of your code.
Format your log messages Evidently and constantly. Include context, for instance timestamps, request IDs, and performance names, so it’s easier to trace difficulties in distributed devices or multi-threaded environments. Structured logging (e.g., JSON logs) could make it even much easier to parse and filter logs programmatically.
In the course of debugging, logs Permit you to monitor how variables evolve, what disorders are satisfied, and what branches of logic are executed—all without the need of halting the program. They’re Primarily useful in output environments in which stepping as a result of code isn’t achievable.
On top of that, use logging frameworks and resources (like Log4j, Winston, or Python’s logging module) that guidance log rotation, filtering, and integration with monitoring dashboards.
Eventually, intelligent logging is about balance and clarity. By using a well-believed-out logging tactic, you are able to decrease the time it's going to take to spot concerns, get further visibility into your applications, and Enhance the Over-all maintainability and trustworthiness of one's code.
Consider Similar to a Detective
Debugging is not merely a technical activity—it is a method of investigation. To effectively recognize and correct bugs, builders will have to method the method just like a detective fixing a thriller. This mentality helps break down complicated concerns into workable sections and abide by clues logically to uncover the foundation cause.
Begin by gathering evidence. Look at the signs of the challenge: error messages, incorrect output, or functionality difficulties. The same as a detective surveys against the law scene, accumulate just as much suitable facts as you may without the need of leaping to conclusions. Use logs, take a look at scenarios, and consumer studies to piece collectively a clear picture of what’s happening.
Next, type hypotheses. Inquire your self: What might be creating this behavior? Have any variations a short while ago been designed to your codebase? Has this situation occurred prior to less than very similar situation? The aim would be to slender down possibilities and recognize opportunity culprits.
Then, take a look at your theories systematically. Endeavor to recreate the challenge inside a managed natural environment. In case you suspect a particular function or part, isolate it and confirm if The difficulty persists. Just like a detective conducting interviews, inquire your code questions and Enable the outcome lead you nearer to the truth.
Pay near focus to small facts. Bugs typically conceal in the minimum expected destinations—like a lacking semicolon, an off-by-a single mistake, or even a race condition. Be extensive and patient, resisting the urge to patch The problem without thoroughly comprehending it. Momentary fixes might cover the real dilemma, just for it to resurface later on.
Last of all, preserve notes on Anything you attempted and figured out. Just as detectives log their investigations, documenting your debugging method can help you save time for long term difficulties and help Other folks have an understanding of your reasoning.
By pondering just like a detective, builders can sharpen their analytical skills, strategy challenges methodically, and become simpler at uncovering concealed difficulties in complex techniques.
Produce Checks
Writing exams is one of the best solutions to help your debugging abilities and All round growth performance. Checks not only assist catch bugs early but additionally serve as a safety Internet that provides you self confidence when building alterations on your codebase. A perfectly-analyzed software is much easier to debug mainly because it more info helps you to pinpoint exactly where and when a problem occurs.
Start with device checks, which deal with unique capabilities or modules. These compact, isolated assessments can promptly expose no matter whether a certain piece of logic is Functioning as anticipated. When a test fails, you immediately know where by to glimpse, noticeably cutting down enough time put in debugging. Unit checks are Primarily handy for catching regression bugs—troubles that reappear right after previously being fastened.
Following, integrate integration checks and conclusion-to-conclude exams into your workflow. These help make sure a variety of areas of your application get the job done collectively smoothly. They’re significantly valuable for catching bugs that take place in complex devices with several components or expert services interacting. If one thing breaks, your tests can inform you which A part of the pipeline unsuccessful and below what disorders.
Composing tests also forces you to definitely think critically regarding your code. To test a attribute correctly, you would like to comprehend its inputs, envisioned outputs, and edge circumstances. This volume of comprehension naturally qualified prospects to raised code construction and much less bugs.
When debugging an issue, composing a failing exam that reproduces the bug could be a robust first step. After the take a look at fails regularly, you may focus on repairing the bug and enjoy your test move when The difficulty is resolved. This strategy makes sure that the same bug doesn’t return Later on.
In a nutshell, crafting tests turns debugging from a annoying guessing activity into a structured and predictable procedure—supporting you capture extra bugs, quicker and a lot more reliably.
Acquire Breaks
When debugging a tough issue, it’s straightforward to become immersed in the challenge—gazing your monitor for several hours, trying Answer soon after solution. But Probably the most underrated debugging resources is just stepping away. Using breaks aids you reset your brain, lessen annoyance, and infrequently see The difficulty from the new point of view.
When you are way too near to the code for way too prolonged, cognitive tiredness sets in. You could possibly start off overlooking evident problems or misreading code that you just wrote just hrs previously. On this state, your brain results in being fewer economical at challenge-fixing. A short walk, a espresso crack, or maybe switching to a distinct activity for 10–15 minutes can refresh your aim. Quite a few builders report locating the root of a dilemma when they've taken time for you to disconnect, letting their subconscious work during the qualifications.
Breaks also aid stop burnout, especially through more time debugging sessions. Sitting down in front of a screen, mentally trapped, is not merely unproductive but also draining. Stepping absent lets you return with renewed Power in addition to a clearer way of thinking. You could suddenly detect a missing semicolon, a logic flaw, or simply a misplaced variable that eluded you ahead of.
In the event you’re trapped, a great general guideline is usually to established a timer—debug actively for 45–sixty minutes, then take a five–10 moment crack. Use that time to maneuver close to, extend, or do one thing unrelated to code. It may well truly feel counterintuitive, Primarily beneath limited deadlines, nevertheless it basically results in a lot quicker and more effective debugging In the long term.
In short, getting breaks is not a sign of weak spot—it’s a sensible method. It presents your brain Area to breathe, enhances your standpoint, and aids you steer clear of the tunnel eyesight that often blocks your progress. Debugging is usually a mental puzzle, and rest is a component of resolving it.
Learn From Each and every Bug
Each individual bug you encounter is much more than simply A short lived setback—it's an opportunity to increase for a developer. Whether or not it’s a syntax error, a logic flaw, or possibly a deep architectural challenge, every one can teach you one thing worthwhile for those who take the time to reflect and evaluate what went Mistaken.
Start out by inquiring you a few important queries after the bug is solved: What brought about it? Why did it go unnoticed? Could it are caught before with improved tactics like device tests, code assessments, or logging? The responses normally expose blind spots within your workflow or knowing and enable you to Create more robust coding behavior relocating forward.
Documenting bugs may also be a great behavior. Maintain a developer journal or maintain a log in which you Take note down bugs you’ve encountered, the way you solved them, and That which you uncovered. After a while, you’ll start to see patterns—recurring challenges or popular faults—you can proactively keep away from.
In crew environments, sharing Everything you've learned from the bug with the peers may be Primarily highly effective. No matter if it’s by way of a Slack message, a brief compose-up, or A fast know-how-sharing session, aiding Other people steer clear of the very same problem boosts workforce effectiveness and cultivates a stronger Discovering tradition.
More importantly, viewing bugs as classes shifts your attitude from frustration to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as critical areas of your development journey. In spite of everything, a number of the most effective developers are not the ones who generate excellent code, but those who continually learn from their problems.
In the end, Every single bug you fix adds a different layer for your ability established. So up coming time you squash a bug, have a instant to reflect—you’ll appear absent a smarter, much more able developer thanks to it.
Conclusion
Strengthening your debugging skills will take time, exercise, and patience — nevertheless the payoff is large. It makes you a more productive, self-assured, and capable developer. The following time you happen to be knee-deep inside of a mysterious bug, keep in mind: debugging isn’t a chore — it’s a chance to be improved at what you do.